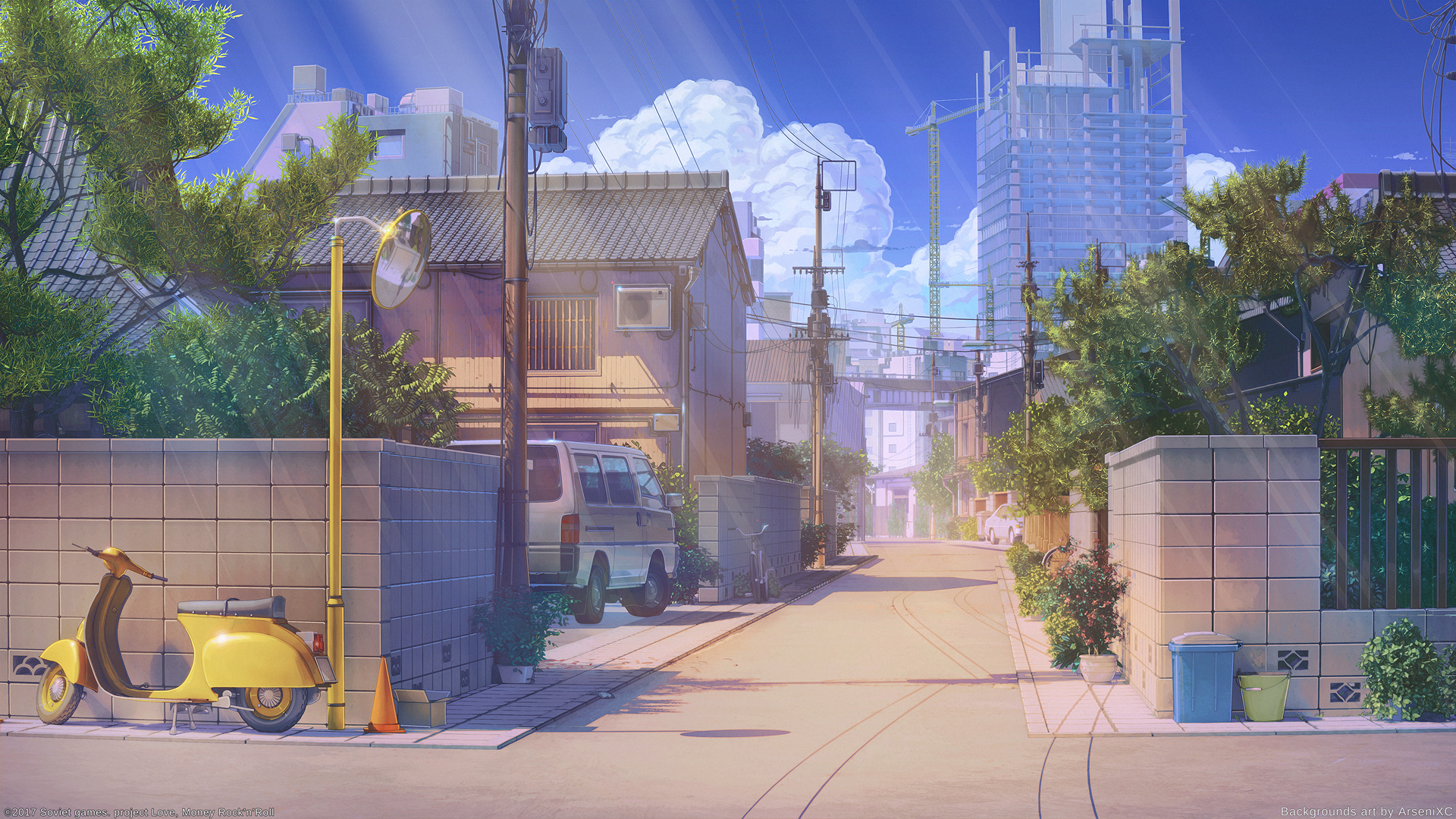
Java – 写入临时文件
Java – 写入临时文件
在某些情况下,可能需要将数据写入 Java 中的临时文件。 因此,让我们快速写下一些示例代码。
使用java.io.BufferedWriter
将数据写入临时文件
此示例是最简单的解决方案,并使用BufferedWriter
编写内容。
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class TemporaryFileExample
{
public static void main(String[] args)
{
File temp;
try
{
temp = File.createTempFile("myTempFile", ".txt");
//write data on temporary file
BufferedWriter bw = new BufferedWriter(new FileWriter(temp));
bw.write("This is the temporary data written to temp file");
bw.close();
System.out.println("Written to Temp file : " + temp.getAbsolutePath());
} catch (IOException e)
{
e.printStackTrace();
}
}
}
使用 NIO 将数据写入临时文件
如果要使用 Java NIO 库,则可以使用Files.write()
方法。
public class TemporaryFileExample
{
public static void main(String[] args)
{
try
{
final Path path = Files.createTempFile("myTempFile", ".txt");
System.out.println("Temp file : " + path);
//Writing data here
byte[] buf = "some data".getBytes();
Files.write(path, buf);
//Delete file on exit
path.toFile().deleteOnExit();
} catch (IOException e)
{
e.printStackTrace();
}
}
}
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果